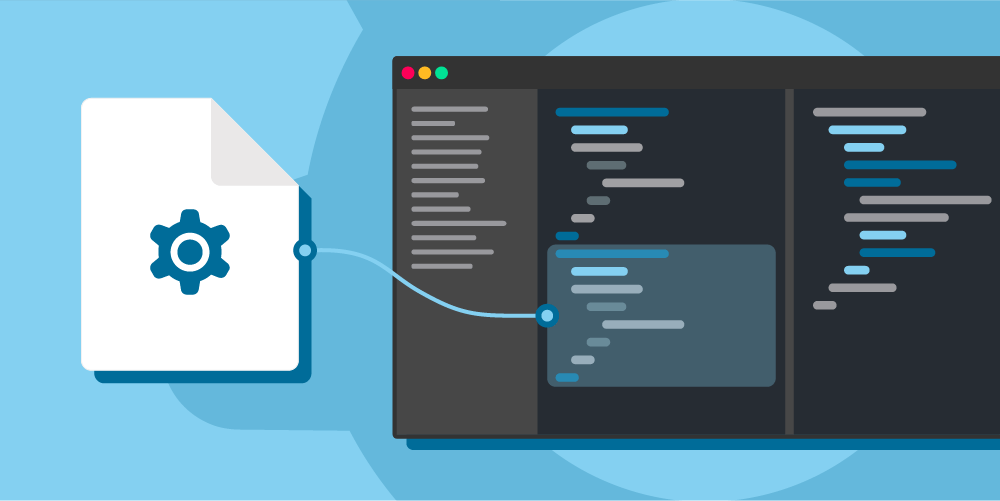
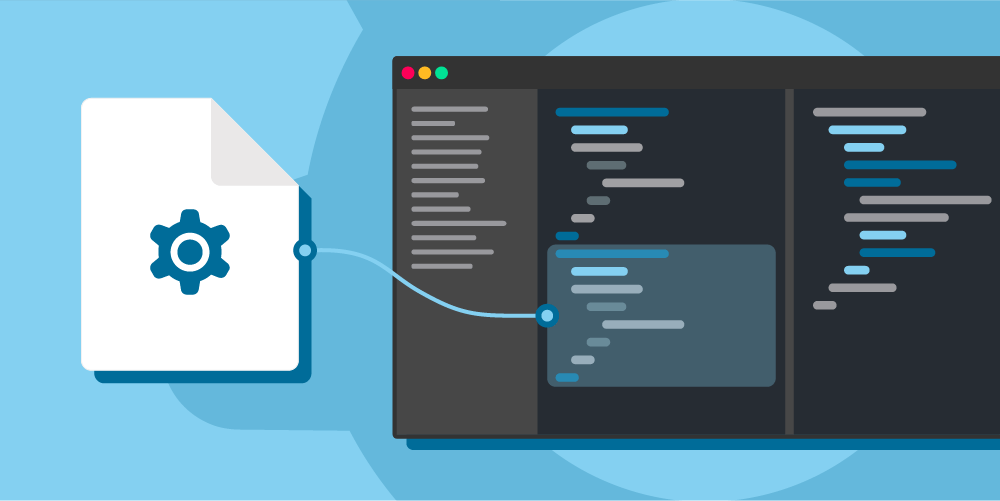
In part two of our guide to Salesforce Files, we broke down the Files data model and the three objects that underpin it: ContentVersion, ContentDocument, and ContentDocumentLink. Now it's time to put your newfound knowledge of Salesforce Files into practice – let's learn how to create and query files in Salesforce using Apex. We'll set you up with code examples to perform CRUD operations, and explain how we use them in our own Drive Connect app.
Creating Salesforce Files with Apex
First things first: let's create a file programatically. In our last post, we went over the order in which the objects that make up a file in Salesforce are created: first, ContentVersion, then, its parent ContentDocument and its child ContentDocumentLink objects. Accordingly, you'll have to follow this same order when creating these objects with Apex. First, you'll create a new ContentVersion record:
Account account = [SELECT Id FROM Account WHERE Name = 'My Account'][0];
ContentVersion contentVersion = new ContentVersion(
Title = 'Content Version Name',
PathOnClient = 'Content Version Name.pdf',
VersionData = 'data',
Origin = 'C'
);
insert contentVersion;
Then, you'll create a ContentDocument linked to that ContentVersion. This example uses the WITH SECURITY_ENFORCED
clause to apply field- and object-level security checks to the SELECT
query – for more on that, check out Salesforce's documentation on the topic.
ContentDocument cd = [
SELECT Id
FROM ContentDocument
WHERE LatestPublishedVersionId = :contentVersion.Id
WITH SECURITY_ENFORCED
];
Finally, you'll create a ContentDocumentLink associated with that ContentDocument:
ContentDocumentLink contentDocumentLink = new ContentDocumentLink(
ContentDocumentId = cd.Id,
LinkedEntityId = account.Id,
ShareType = 'V'
);
insert contentDocumentLink;
Querying Salesforce Files with Apex
Next, here's how to query the ContentVersion object with Apex. Here, versionId represents a value you retrieve or pass to a method.
ContentVersion contentVersion = [
SELECT Id, Title
FROM ContentVersion
WHERE Id = :versionId
];
Deleting Salesforce Files with Apex
Finally, here's a snippet of code you can use to delete a file. This function will identify and delete the ContentDocument that houses all versions of a given file, which in turn deletes its child records and all links associated with it.
delete [
SELECT Id
FROM ContentDocument
WHERE Id = :ContentDocumentId
];
These are just some of the tricks we use in Drive Connect, allowing users to link, view, and create Google documents without ever leaving Salesforce. Our all-in-one solution for integrating Salesforce with Google Drive yourself is free for 14 days – try it yourself.
Get Started with Drive Connect
You can use the app for 14 days with no credit card required.